pragma solidity 0.4.18;
import “./Vehicle.sol”;
contract VehicleOwner {
address public owner;
mapping(bytes32 => address) public vehicles;
event NewVehicleAdded(address indexed newVehicle, uint256 timestamp);
function VehicleOwner() public {
owner = msg.sender;
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
require(msg.sender == owner);
_;
}
function createNewVehicle(string model, string make, bytes32 vin) public onlyOwner {
address newVehicle = new Vehicle(model, make, vin);
vehicles[vin] = newVehicle;
NewVehicleAdded(newVehicle, now);
}
}
So, let’s go line and by line and understand what is happening here. Code: pragma solidity 0.4.18; Analysis: Specifies the version of the compiler used. In this 0.4.18 Code: import “./Vehicle.sol”; Analysis: Imports the smart contract which is used to represent new vehicles. Code: contract VehicleOwner { Analysis: Declares the vehicle owner contract. Code:address public owner;mapping(bytes32 => address) public vehicles; Analysis: This is where we flesh out our contract. This first variable calls the owner and represents the Ethereum that created any given instance of the VehicleOwner contract.The second one, called vehicles, will be used to store a list of the vehicles owned by the owner, by assigning their contracts’ addresses to the provided vehicle identification numbers. Code:function VehicleOwner() public {owner = msg.sender;} Analysis: See how the function has the same name as the contract? This is because this particular function is a constructor. The only function that it does is to assign the address that called the function as the contract owner. Code:modifier onlyOwner() {require(msg.sender == owner);_;} Analysis: This function modifier is used make sure that only the contract owner has access to the contract. See that “_”? This yields for the body of the function to which the modifier is later applied. Code:function createNewVehicle(string model, string make, bytes32 vin) public onlyOwner {address newVehicle = new Vehicle(model, make, vin);vehicles[vin] = newVehicle;NewVehicleAdded(newVehicle, now);} Analysis: This creates a new contract on the blockchain which is a representation of a new vehicle. The vehicle contract’s constructor receives three properties: model, make, and vin, the latter of which can be used to identify that particular vehicle.Creating a new contract returns its newly assigned address. In the function, using the vehicle’s mapping, we bind the given vin to that address. Finally, the function broadcasts a new event, passing in the address and the current timestamp.Code Example #2contract BasicIterator
{
address creator; // reserve one “address”-type spot
uint8[10] integers; // reserve a chunk of storage for 10 8-bit unsigned integers in an array
function BasicIterator()
{
creator = msg.sender;
uint8 x = 0;
//Section 1: Assigning values
while(x < integers.length) {
integers[x] = x;
x++;
} }
function getSum() constant returns (uint) {
uint8 sum = 0;
uint8 x = 0;
//Section 2: Adding the integers in an array.
while(x < integers.length) {
sum = sum + integers[x];
x++;
}
return sum;
}
// Section 3: Killing the contract
function kill()
{
if (msg.sender == creator)
{
suicide(creator);
}
}
}
So, let’s analyse.
Section 1: Assigning Values
In the first step we are filling up an array called “integers” which takes in 10 8-bit unsigned integers. The way we are doing it is via a while loop. Let’s look at what is happening inside the while loop.
while(x < integers.length) {
integers[x] = x;
x++;
}
Remember, we have already assigned a value of “0” to the integer x. The while loop goes from 0 to integers.length. Integers.length is a function which returns the max capacity of the array. So, if we decided that an array will have 10 integers, arrayname.length will return a value of 10. In the loop above, the value of x goes from 0 – 9 (<10) and assigns the value of itself to the integers array as well. So, at the end of the loop, integers will have the following value:0,1,2,3,4,5,6,7,8,9.Section 2: Adding the array contentInside the getSum() function we are going to add up the contents of the array itself. The way its going to do it is by repeating the same while loop as above and using the variable “sum” to add the contents of the array.Section 3: Killing the contractThis function kills the contract and sends the remaining funds in the contract back to the contract creator.So this should give you a good idea of what solidity contracts look like and what kind of code breakdown you should expect from your prospects.What’s the difference between Ether and Gas?This is another core concept that your developers should be familiar with.Ether is the main token in the ecosystem. It is what incentivizes the players to carry out their end of the smart contract.Gas is the amount of fuel that is required to fulfill all the needs of a given contract.When someone submits a smart contract, it has a pre-determined gas value. When the contract is executed each and every step of the contract requires a certain amount of gas to execute.This can lead to two scenarios:The gas required is more than the limit set. If that’s the case then the state of the contract is reverted back to its original state and all the gas is used up.The gas required is less than the limit set. If that’s the case, then the contract is completed and the leftover gas is given over to the contract setter.The following is a graph that shows the average gas price in Wei.Image Credit: EtherscanGas is the lifeblood of Ethereum.All the transactions in Ethereum are validated by the miners. Basically, they have to manually put each and every transaction into the blocks that they have mined for the transaction to be validated. In exchange for their services, they collect a certain amount of transaction fees.Usually, smart contracts with high gas fees are given preference because the miners have the chance to collect higher fees there. The fee collected though is still pretty nominal as compared to bitcoin.This graph here compares the transaction fees of Bitcoin to Ethereum.Image Credit: BitinfochartsIn fact, as you can see, in this transaction of 0.01 Ether only 0.00000000000002 Ether was collected as transaction fees which is <$0.000001.Image Credit: EtherscanSo, as you can see, the miners in Ethereum, writing, collect very nominal transaction fees. Obviously collect transaction fees is a secondary role for there miners, their main job is to…well…mine!Questions and AnswersSo, distilling all this knowledge, let’s zero in on some specific questions that you can ask.Q) How is the contract constructor defined?A) The constructor is defined as a function, named exactly the same as the contract. Q) Where are events logged in Ethereum and what’s their purpose?A) Logs are the events emitted by the contracts. These are parts of their transactions’ receipts and the results of the LOG opcodes which are executed on the Ethereum Virtual Machine (EVM).The events are primarily used to communicate with front ends or as cheap data storage. Because the return values of transactions are only the transactions hashed, because it takes a bit of time for the blockchain to reach consensus and validate the transactions, by mining them into new blocks. By emitting events and having front ends listen (watch) for those events, efficient communication is achieved.Q) What are the mappings?A) Mapping is equivalent to a dictionary or a map in other programming languages. It’s key-to-value storage. Q) What is the purpose of modifiers?A) As the name suggests; they modify the functions that use them. However, the conditions of the modifier must be met before the function gets executed. If not, then the modifiers throws an error. Q) What are Ethereum libraries?A) Ethereum libraries help in the isolation of integral pieces of logic. They are a group of packages built for use on blockchains utilizing the Ethereum Virtual Machine (EVM). All libraries are deployed and linkable in your smart contracts. They can be called via DELEGATECALL. Q) Why does it cost money to invoke a method on a Smart Contract?A) When methods get invoked, they change the state of the blockchain. Since the operation requires gas, it costs money. Where can you find great developers?It is hard to find great developers in “traditional places” like LinkedIn and Google. However, Reddit, GitHub etc. are great places to find these developers.Plus, there is one more thing. Since this is such a niche talent, you should be open to the fact that they might be in your city or even your own country. You should make provisions for remote location jobs, especially if you are looking for the cream of the crop.It may be a pain, but this is one of those “quality over quantity” things.How To Hire Ethereum Developers: ConclusionWhen you are interviewing Ethereum developers, you must keep one thing in mind. It is not necessary that they answer all the questions thoroughly. What matters is their passion and whether they were able to specifically answer the questions which pertain to their job and role.Anyway, this guide should help you zero in on amazing blockchain developers. Just one final word of advice. Please do not compromise on the quality of the developers. Remember, quality will always trump quantity.
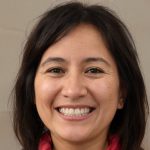
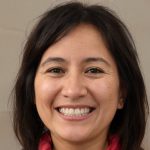
I have over 10 years of experience in the Crypto industry. I have written numerous articles on Cryptocurrency, Blockchain technology, and Bitcoin. I’m a well-known speaker in the Crypto community and have spoken at many conferences worldwide.